6a84d58ee6
PHP Parser written in Go
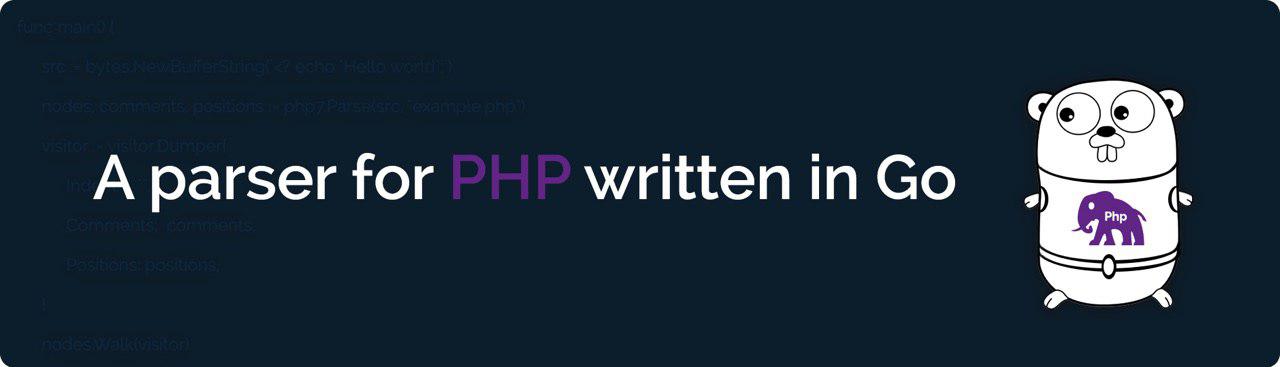
This project uses goyacc and ragel tools to create PHP parser. It parses source code into AST. It can be used to write static analysis, refactoring, metrics, code style formatting tools.
Try it online: demo
Features:
- Fully support PHP 5 and PHP 7 syntax
- Abstract syntax tree (AST) representation
- Traversing AST
- Resolving namespaced names
- Parsing syntax-invalid PHP files
- Saving and printing free-floating comments and whitespaces
Who Uses
VKCOM/noverify - NoVerify is a pretty fast linter for PHP
quasilyte/phpgrep - phpgrep is a tool for syntax-aware PHP code search
Usage example
package main
import (
"fmt"
"os"
"github.com/z7zmey/php-parser/php7"
"github.com/z7zmey/php-parser/visitor"
)
func main() {
src := []byte(`<? echo "Hello world";`)
parser := php7.NewParser(src, "7.4")
parser.Parse()
for _, e := range parser.GetErrors() {
fmt.Println(e)
}
visitor := visitor.Dumper{
Writer: os.Stdout,
Indent: "",
}
rootNode := parser.GetRootNode()
rootNode.Walk(&visitor)
}
Roadmap
- Control Flow Graph (CFG)
- PhpDocComment parser
- Stabilize api
Install
go get github.com/z7zmey/php-parser
CLI
php-parser [flags] <path> ...
flag | type | description |
---|---|---|
-p | bool | print filepath |
-d | string | dump format: [custom, go, json, pretty-json] |
-r | bool | resolve names |
-ff | bool | parse and show free floating strings |
-prof | string | start profiler: [cpu, mem, trace] |
-php5 | bool | parse as PHP5 |
Dump AST to stdout.
Namespace resolver
Namespace resolver is a visitor that resolves nodes fully qualified name and saves into map[node.Node]string
structure
- For
Class
,Interface
,Trait
,Function
,Constant
nodes it saves name with current namespace. - For
Name
,Relative
,FullyQualified
nodes it resolvesuse
aliases and saves a fully qualified name.
Description
Languages
Go
92.7%
Yacc
6%
Ragel
1.3%