.github | ||
cmd/php-parser | ||
internal | ||
pkg | ||
.editorconfig | ||
.gitattributes | ||
.gitignore | ||
.golangci.yml | ||
CHANGELOG.md | ||
CODE_OF_CONDUCT.md | ||
CONTRIBUTING.md | ||
go.mod | ||
go.sum | ||
LICENSE | ||
Makefile | ||
parser.jpg | ||
README.md |
This is a merge of laytan/php-parser and jeremybobbin/php-parser, fixing some crashes in VKCOM php-parse, which in itself is a fork of z7zmey parser that adds PHP 8 support.
PHP Parser written in Go
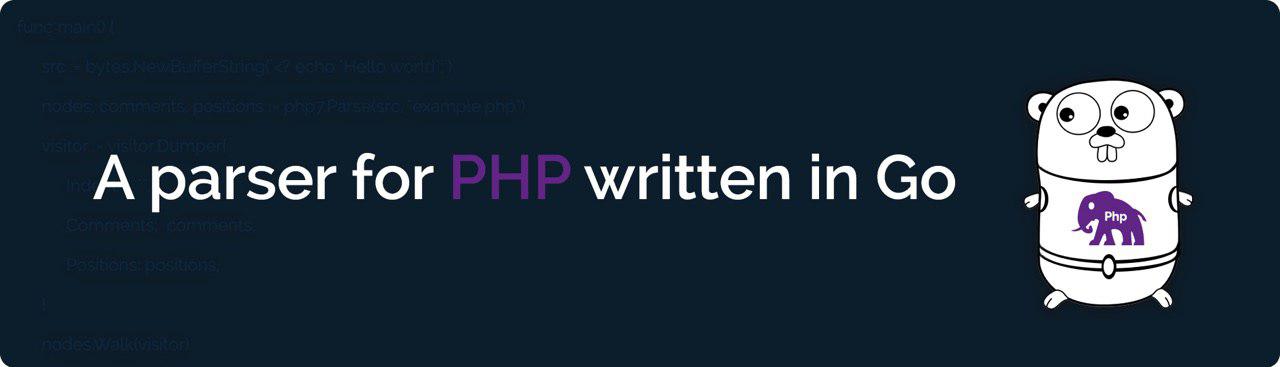
This project uses goyacc and ragel tools to create PHP parser. It parses source code into AST. It can be used to write static analysis, refactoring, metrics, code style formatting tools.
Features
- Fully support PHP 5, PHP 7 and PHP 8.0-8.2 syntax
- Abstract syntax tree (AST) representation
- Traversing AST
- Resolving namespace names
- Parsing syntax-invalid PHP files
- Saving and printing free-floating comments and whitespaces
Who Uses
- VKCOM/noverify — pretty fast linter for PHP
- VKCOM/nocolor — architecture validation tool for PHP based on the concept of colored functions
- quasilyte/phpgrep — tool for syntax-aware PHP code search
Usage example
package main
import (
"log"
"os"
"github.com/VKCOM/php-parser/pkg/conf"
"github.com/VKCOM/php-parser/pkg/errors"
"github.com/VKCOM/php-parser/pkg/parser"
"github.com/VKCOM/php-parser/pkg/version"
"github.com/VKCOM/php-parser/pkg/visitor/dumper"
)
func main() {
src := []byte(`<?php echo "Hello world";`)
// Error handler
var parserErrors []*errors.Error
errorHandler := func(e *errors.Error) {
parserErrors = append(parserErrors, e)
}
// Parse
rootNode, err := parser.Parse(src, conf.Config{
Version: &version.Version{Major: 8, Minor: 0},
ErrorHandlerFunc: errorHandler,
})
if err != nil {
log.Fatal("Error:" + err.Error())
}
if len(parserErrors) > 0 {
for _, e := range parserErrors {
log.Println(e.String())
}
os.Exit(1)
}
// Dump
goDumper := dumper.NewDumper(os.Stdout).
WithTokens().
WithPositions()
rootNode.Accept(goDumper)
}
Install
go get github.com/VKCOM/php-parser/cmd/php-parser
CLI
php-parser [flags] <path> ...
flag | type | description |
---|---|---|
-p |
bool |
Print file paths |
-e |
bool |
Print errors |
-d |
bool |
Dump AST in Golang format |
-r |
bool |
Resolve names |
--pb |
bool |
Print AST back into the parsed file |
--time |
bool |
Print execution time |
--prof |
string |
Start profiler: [cpu, mem, trace] |
--phpver |
string |
PHP version (default: 8.0) |
Namespace resolver
Namespace resolver is a visitor that resolves nodes fully qualified name and saves into map[ast.Vertex]string
structure
- For
Class
,Interface
,Trait
,Enum
,Function
,Constant
nodes it saves name with current namespace. - For
Name
,Relative
,FullyQualified
nodes it resolvesuse
aliases and saves a fully qualified name.